If you read my previous post on how to create WooCommerce Order programmatically, you will be interested in this new post on how to create WooCommerce product programmatically. In most cases this is required when you are creating a WooCommerce plugin that requires such a functionality.
To create WooCommerce product programmatically, you need to understand that a WooCommerce product is a post since the Products are essentially custom post types.
WooCommerce Create Product Programmatically
To create product programmatically in WooCommerce you just need to create the post with the product details using the wp_insert_post() function indicating that the post you insert into the WordPress database should be in the product custom post type.
The most important part of the logic is where you specify the custom post type in the wp_insert_post() array
. In this case it should be the product – post_type' => 'product'
,
The code below is a function that you can add to the functions.php or your plugin files to create product programmatically:
<?php function create_Products_Programmatically(){ // Set number of products to create $number_of_products = 100; for ($i=1; $i <= $number_of_products; $i++) { // First we create the product post so we can grab it's ID $post_id = wp_insert_post( array( 'post_title' => 'Product ' . $i, 'post_type' => 'product', 'post_status' => 'publish' ) ); // Then we use the product ID to set all the posts meta wp_set_object_terms( $post_id, 'simple', 'product_type' ); // set product is simple/variable/grouped update_post_meta( $post_id, '_visibility', 'visible' ); update_post_meta( $post_id, '_stock_status', 'instock'); update_post_meta( $post_id, 'total_sales', '0' ); update_post_meta( $post_id, '_downloadable', 'no' ); update_post_meta( $post_id, '_virtual', 'yes' ); update_post_meta( $post_id, '_regular_price', '' ); update_post_meta( $post_id, '_sale_price', '' ); update_post_meta( $post_id, '_purchase_note', '' ); update_post_meta( $post_id, '_featured', 'no' ); update_post_meta( $post_id, '_weight', '11' ); update_post_meta( $post_id, '_length', '11' ); update_post_meta( $post_id, '_width', '11' ); update_post_meta( $post_id, '_height', '11' ); update_post_meta( $post_id, '_sku', 'SKU11' ); update_post_meta( $post_id, '_product_attributes', array() ); update_post_meta( $post_id, '_sale_price_dates_from', '' ); update_post_meta( $post_id, '_sale_price_dates_to', '' ); update_post_meta( $post_id, '_price', '11' ); update_post_meta( $post_id, '_sold_individually', '' ); update_post_meta( $post_id, '_manage_stock', 'yes' ); // activate stock management wc_update_product_stock($post_id, 100, 'set'); // set 1000 in stock update_post_meta( $post_id, '_backorders', 'no' ); } }
The function in the code snippet above creates 100 products and you can adjust the number to create the number of products you wish. You can also hook this function as a callback function to specific event that will trigger the creation of the products programmatically.
For example you can hook this on initialization or you can hook it on plugin or theme activation hooks and the products will be created when the specific hook event action occurs.
The last part of the function updates the post meta for the product.
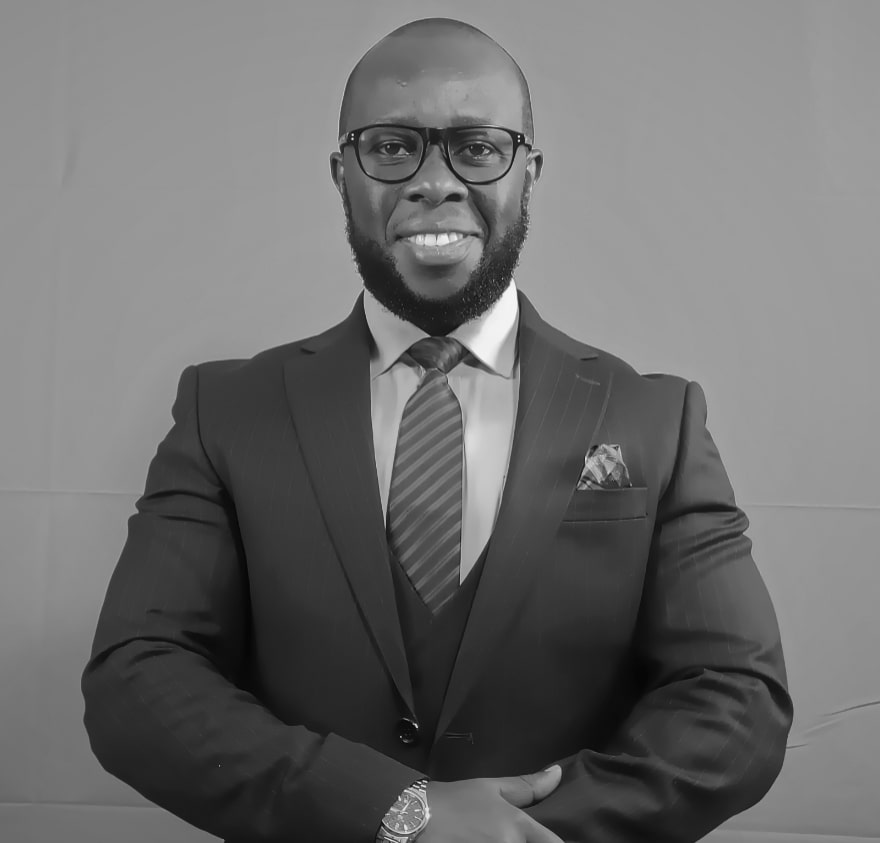
Joe is an experienced full-stack web developer with a decade of industry experience in the LAMP & MERN stacks, WordPress, WooCommerce, and JavaScript – (diverse portfolio). He has a passion for creating elegant and user-friendly solutions and thrives in collaborative environments. In his spare time, he enjoys exploring new tech trends, tinkering with new tools, and contributing to open-source projects. You can hire me here for your next project.
Similar Articles
- How to Set WooCommerce Storefront Thumbnail Sizes
- How Add Text Before the Price in WooCommerce » Add Text Before Price
- How to Get Current Product Category Name in WooCommerce
- How to Setup WooCommerce Storefront Blog
- How to Check if User is Logged In WordPress
- How to Hide Tax Label In WooCommerce
- How to Clear Cart on Logout In WooCommerce
- How to Create WooCommerce Login Logout Shortcode
- How to Hide Prices From Google In WooCommerce
- How to Hide Price If Zero In WooCommerce
- How to Create Order Programmatically WooCommerce
- How to Remove Product Category Title WooCommerce
- How to Hide Out of Stock Variations in WooCommerce
- How to Add WooCommerce Product from Frontend
- WooCommerce Logout PHP Snippet to Create Logout Button
- WooCommerce Mobile Checkout Optimization Quick Guide
- How to Create WooCommerce Storefront Child Theme [Complete Guide]
- How to Change Storefront Number of Products Per Row
Comments are closed.