When you are creating a WooCommerce plugin that creates and manages WooCommerce orders, you may want to create order programmatically.
If this is something you have in mind and want to see an example of how you can get this done, I want to share with you a good example of a function I have used before in my plugins to create order programmatically in WooCommerce. I also shared in a previous post how to Change WooCommerce product price programmatically
WooCommerce Create Order Programmatically
We can use a function to create the order programmatically that sets the order number first, loops through the orders and sets the address for the automatic order.
We can then use the wc_create_order() function to create order programmatically and set the products in the order and the order status to complete for the order to be completed.
I have created an example function below with all the necessary code that you can repurpose to create order programmatically in WooCommerce.
Ideally, you should add this code to the functions.php
or your plugin files and then call the function where to need it. You can also adjust the $number_of_orders
variable to change the number of orders that are created when the function is called.
The following is the code that you can use to create automatic orders in WooCommerce :
<?php function create_Orders_Programmatically(){ // Set numbers of orders to create $number_of_orders = 50; for ($i=0; $i < $number_of_orders; $i++) { global $woocommerce; // Get a random number of products, between 1 and 10, for the order $products = get_posts( array( 'post_type' => 'product', 'posts_per_page' => rand(1,10), 'orderby' => 'rand' ) ); // Set order address $address = array( 'first_name' => 'Joe ' . rand(1,200), 'last_name' => 'Njenga', 'company' => 'njengah.com', 'email' => 'joe@example.com', 'phone' => '894-672-780', 'address_1' => '123 Main st.', 'address_2' => '100', 'city' => 'Nairobi', 'state' => 'Nairobi', 'postcode' => '00100', 'country' => 'KE' ); // Now we create the order $order = wc_create_order(); // Add products randomly selected above to the order foreach ($products as $product) { $order->add_product( wc_get_product($product->ID), 1); // This is an existing SIMPLE product } $order->set_address( $address, 'billing' ); $order->calculate_totals(); $order->update_status("Completed", 'Imported order', TRUE); }
As you can see in the code above, its possible to create programmatic orders using the steps I highlighted above. You can adjust the variable in each case to suit your needs.
In the case of this example I am creating 50 orders and this logic can be extended further to add more specific products that can have automatic orders.
Conclusion
In this post, I have highlighted how you can create WooCommerce orders programmatically using a function that sets the order variable, sets address and creates the WooCommerce order. I hope this code helps you create the order programmatically that suits your needs. If you need further help in getting this code to work, you should get in touch with me.
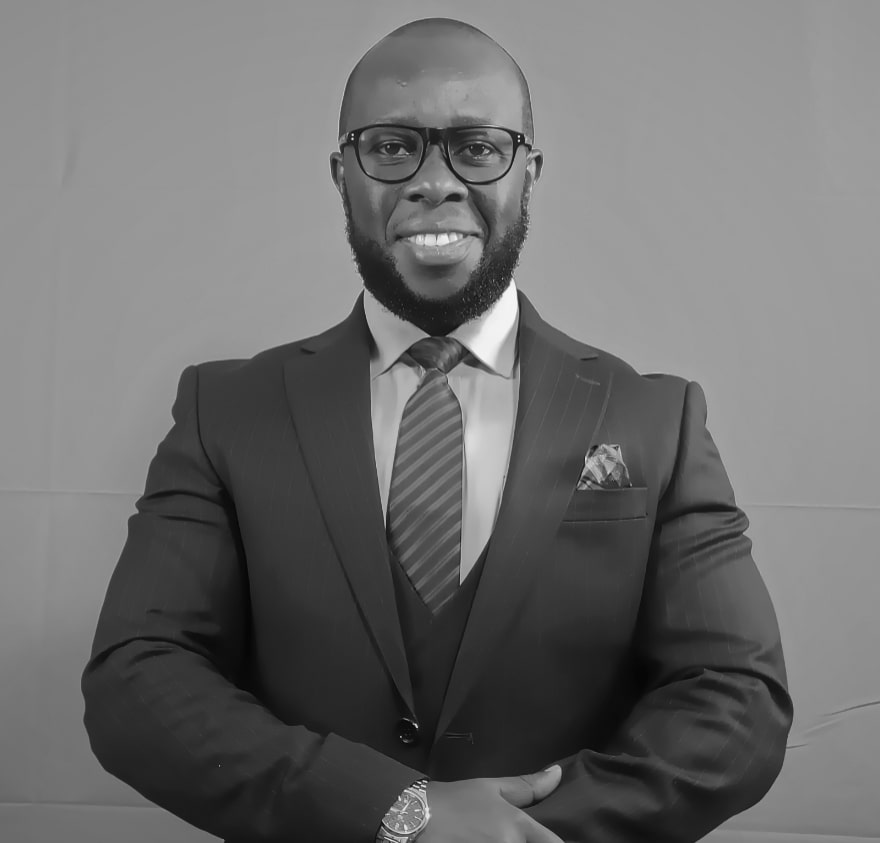
Joe is an experienced full-stack web developer with a decade of industry experience in the LAMP & MERN stacks, WordPress, WooCommerce, and JavaScript – (diverse portfolio). He has a passion for creating elegant and user-friendly solutions and thrives in collaborative environments. In his spare time, he enjoys exploring new tech trends, tinkering with new tools, and contributing to open-source projects. You can hire me here for your next project.
Similar Articles
- WooCommerce Checkout Optimization Quick Guide
- How to Hide Prices From Google In WooCommerce
- How to Hide Tax Label In WooCommerce
- How to Change Product Price Programmatically in WooCommerce
- How to Create WooCommerce Login Logout Shortcode
- How to Setup WooCommerce Storefront Blog
- How to Add Custom Order Status in WooCommerce
- How to Remove Email Footer Text ‘Built With WooCommerce’
- How to Redirect a WordPress Page Without Plugins?
- How to Check If Plugin is Active In WordPress [ 3 WAYS ]
- How to Display Category Name in WordPress Using a Practical Example
- How to Select All Except Last Child In CSS » CSS Not Last Child Example
- How to Create WooCommerce Storefront Child Theme [Complete Guide]
- How to Clear Cart on Logout In WooCommerce
- How to Check if User is Logged In WordPress
- How to Auto Approve Orders in WooCommerce
- WooCommerce Login Redirect Hook Explained with Example
- How to Hide Customer Order Email For Free Orders WooCommerce