When you are creating a WordPress theme you need to display the category name, especially in the WordPress theme homepage. On the home page, you have layouts with featured posts from different WordPress categories. If you want to know how to display category name in WordPress; this post will guide you and I will share with you the code to use to display any category in WordPress.
How to Display Category Name
The WordPress function that you should use to retrieve the categories is get_the_category(). This function is used to get all the categories in your WordPress site. The general expression of this function is as follows :
get_the_category( int $id = false )
As you can see it takes only one parameter and the parameter is the $id
which is equivalent to the current post ID. You should pass the id to the function and this is an integer and by default this parameter is FALSE
.
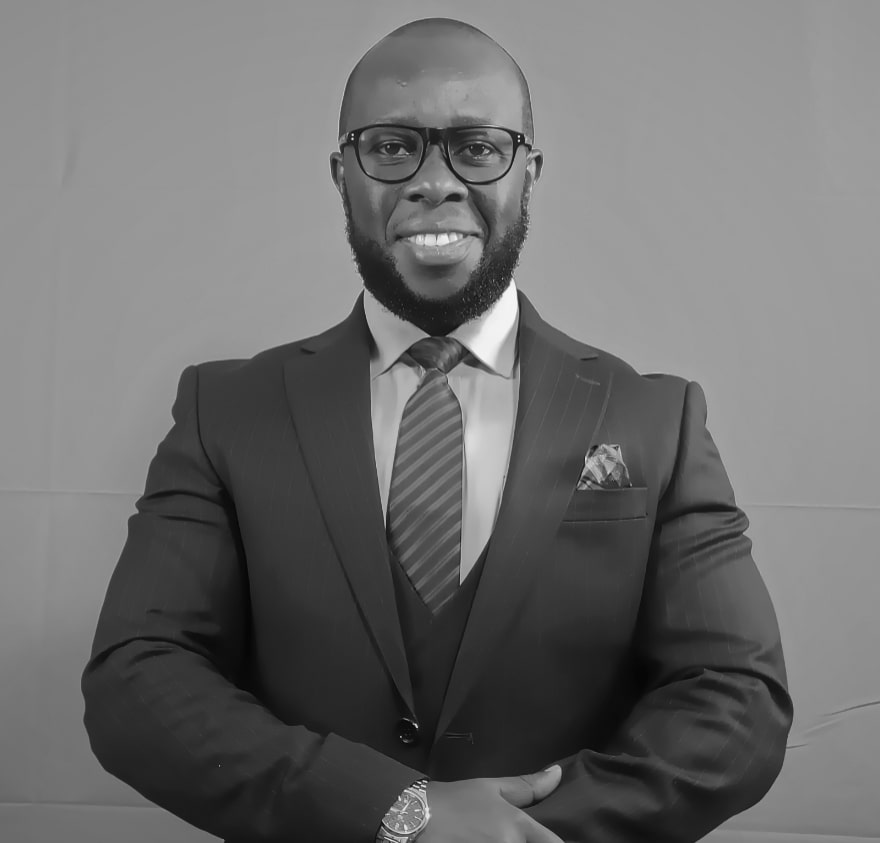
Joe is an experienced full-stack web developer with a decade of industry experience in the LAMP & MERN stacks, WordPress, WooCommerce, and JavaScript – (diverse portfolio). He has a passion for creating elegant and user-friendly solutions and thrives in collaborative environments. In his spare time, he enjoys exploring new tech trends, tinkering with new tools, and contributing to open-source projects. You can hire me here for your next project.
Example of How to Display Category Name
The function above returns a category object with all the information about a specific category. I have created a quick code snippet that gets the current post ID from the global post object.
The current post ID is then passed to the get_the_category()
function. A print_r
function is used to display the data for visibility.
add_action('wp_head', 'test_category_name_display'); function test_category_name_display(){ global $post; $cat = get_the_category($post->ID); //Print_r to test what it returns print('<pre>'); print_r($cat); print('</pre>'); }
This code will display on the header the WP_Term Object
with all the details about the category. For example, the following is the data of the current category on my tutorial example :
Array ( [0] => WP_Term Object ( [term_id] => 46 [name] => Cool Category [slug] => cool-category [term_group] => 0 [term_taxonomy_id] => 46 [taxonomy] => category [description] => [parent] => 0 [count] => 1 [filter] => raw [cat_ID] => 46 [category_count] => 1 [category_description] => [cat_name] => Cool Category [category_nicename] => cool-category [category_parent] => 0 ) )
Here we have all the data that we need and we can easily pick whatever we need from the array. In this case, we need to display the name of the category that is at this point in the array :
You can now access the category and display it using the following code that I have modified and removed the print_r()
replacing it with the code to display the category name.
/** * Display Category in WordPress */ add_action('wp_head', 'test_category_name_display'); function test_category_name_display(){ global $post; $cat = get_the_category($post->ID); if ( ! empty( $cat ) ) { echo esc_html( $cat [0]->cat_name ); } }
When you add this code to the functions.php and you visit the post you should see the category displayed on the header as shown on the image below :
Comments are closed.