If you want to get the post id by slug in WordPress, you can do so using a function that passes the slug as a parameter and returns the post ID or Page ID.
This may sound like a complicated WordPress function but it is very straightforward and easy to implement in your theme or a custom plugin.
Get Post ID / Slug
You can create the function to get the post id from the slug as follows :
/** * Function to get post ID By slug */ function get_post_id_by_slug($slug) { $post = get_page_by_path($slug); if ($post) { return $page->ID; } else { return null; } }
This function can be added to the functions.php. When you add this function; you can get the post ID by passing the slug , as you call the function as follows:
get_post_id_by_slug('some-slug');
How to Get Post ID / Slug Example
Open your functions.php and add the code below that we have created that will retrieve the post ID by the slug. In the header (‘wp_head’), I want to call this function to display the post ID from the slug I will add as a string and the post type. I will create another action hook to call this and display the results on the header:
/** * Function to get post ID By slug */ function get_id_by_slug($page_slug, $slug_page_type = 'page') { $find_page = get_page_by_path($page_slug, OBJECT, $slug_page_type); if ($find_page) { return $find_page->ID; } else { return null; } }
Second Code Snippet to Display the Page ID in the Header
/** * Testing by Display to Header */ add_action('wp_head' , 'test_function'); function test_function(){ //calling the function here to display the page ID in the header echo get_id_by_slug('login-page','page'); }
As you can see in these two code snippets we are getting the page by the slug and retrieving the IDand on the second code snippet we are displaying the ID as shown in the image below to just prove it works :
As you can see in the code above we have extended the first function to add the support for the custom post type parameter and the general code can be as follows :
/** * Function to Get Post ID by slug extended for Custom Post Types */ function get_post_id_by_slug($post_slug, $slug_post_type = 'post') { $post = get_page_by_path($post_slug, OBJECT, $slug_post_type); if ($post) { return $post->ID; } else { return null; } } // Usage: get_post_id_by_slug('some-post-slug','some-post-type');
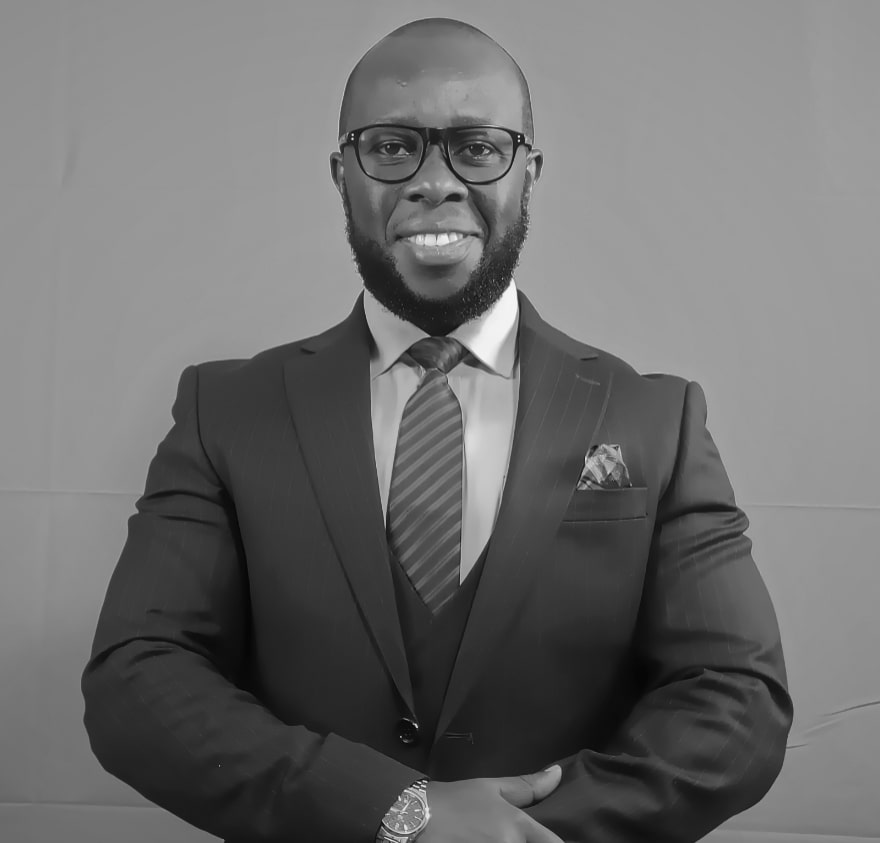
Joe is an experienced full-stack web developer with a decade of industry experience in the LAMP & MERN stacks, WordPress, WooCommerce, and JavaScript – (diverse portfolio). He has a passion for creating elegant and user-friendly solutions and thrives in collaborative environments. In his spare time, he enjoys exploring new tech trends, tinkering with new tools, and contributing to open-source projects. You can hire me here for your next project.
Conclusion
In this post, we have illustrated a practical example of how you can get a post id slug in WordPress. It is important to remember that at the core of these code snippets, we are using the default WordPress function – get_page_by_path().
Comments are closed.